When interacting with Android Apps, share sheets are a common component that we’ll interact with when sharing content with other apps and contacts. As developers, we can improve the usability of these share sheets by utilising features such as adding previews for content being shared, as well as implementing direct share targets to commonly interacted contacts/apps. Alongside these features, Android 14 adds support for custom-chooser actions which allows us to further customise these sheets. In this blog post, we’ll explore these custom actions and learn how we can add them to our own share sheets.
Why not sponsor this blog and reach thousands of developers worldwide?
On Android 14, Custom Actions provides our users with direct access to common functionality when sharing content from our application.
For example, let’s say that our application allows users to share files via the share sheet. In this case, we’ll show some custom actions that allow the users to create links to be shared, or directly share that file to a different folder from where it is currently stored.
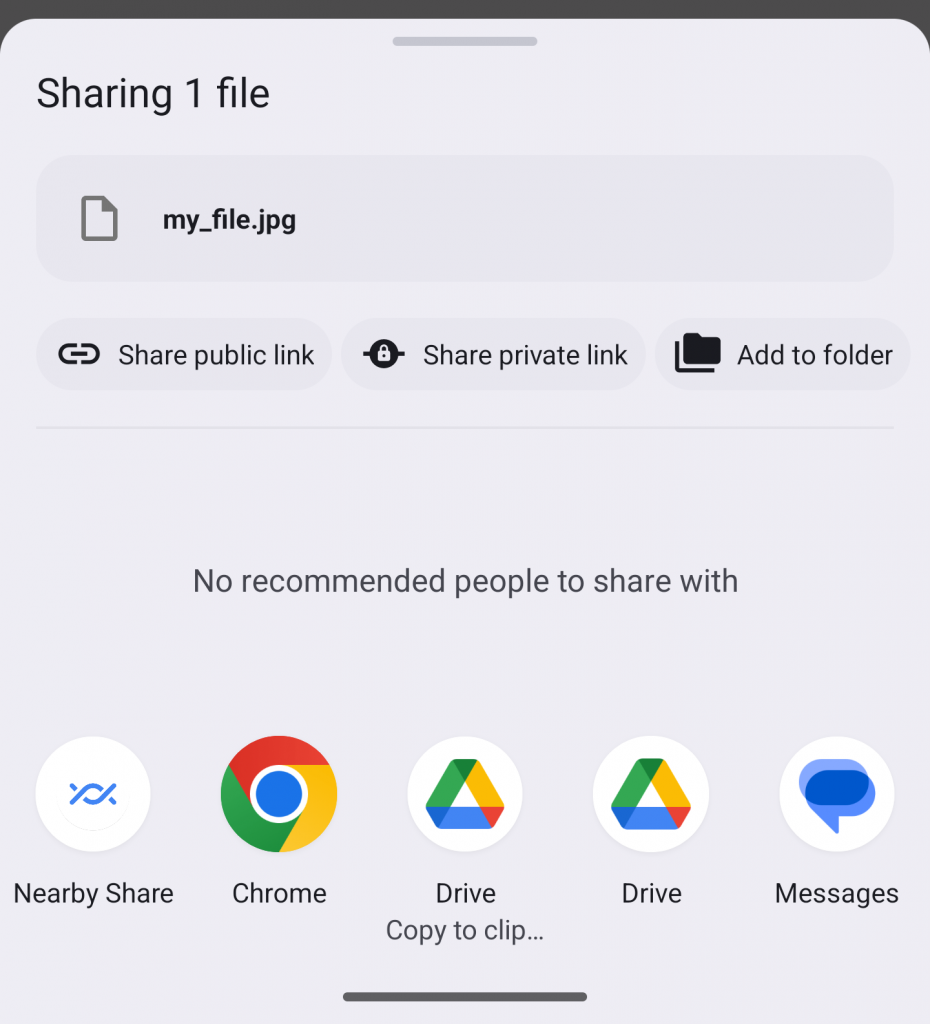
Share sheets in mobile actions are extremely common user flows, so providing direct access to these actions greatly reduces the friction for performing certain things in our apps.
To create these custom actions we can utilise the ChooserAction class – this class represents an individual action that is to be displayed within the share sheet. When it comes to these actions, there are three key pieces of information that we need to provide each with:
- icon – the icon to be displayed alongside the action
- label – the label used to describe the intent of the action
- intent – the pending intent to be associated with the action
When it comes to creating a chooser action, we can utilise the Builder class to instantiate an instance of an action.
ChooserAction.Builder(
Icon.createWithResource(this, R.drawable.baseline_insert_link_24),
"Share public link",
PendingIntent.getBroadcast(
this,
1,
Intent(Intent.ACTION_VIEW),
PendingIntent.FLAG_IMMUTABLE or PendingIntent.FLAG_CANCEL_CURRENT
)
).build()
When it comes to providing our actions to our share sheet, we need to provide an array of actions to our share intent using the Intent.EXTRA_CHOOSER_CUSTOM_ACTIONS key.
shareIntent.putExtra(Intent.EXTRA_CHOOSER_CUSTOM_ACTIONS, customActions)
If we piece this together with a complete example, we could see a share sheet with multiple custom actions looking like the following:
val sendIntent = Intent(Intent.ACTION_SEND)
.setType("text/plain")
.putExtra(Intent.EXTRA_STREAM, Uri.parse("path_to_file"))
val shareIntent = Intent.createChooser(sendIntent, null)
val customActions = arrayOf(
ChooserAction.Builder(
Icon.createWithResource(this, R.drawable.baseline_insert_link_24),
"Share public link",
PendingIntent.getBroadcast(
this,
1,
Intent(Intent.ACTION_VIEW),
PendingIntent.FLAG_IMMUTABLE or PendingIntent.FLAG_CANCEL_CURRENT
)
).build(),
ChooserAction.Builder(
Icon.createWithResource(this, R.drawable.baseline_private_connectivity_24),
"Share private link",
PendingIntent.getBroadcast(
this,
1,
Intent(Intent.ACTION_VIEW),
PendingIntent.FLAG_IMMUTABLE or PendingIntent.FLAG_CANCEL_CURRENT
)
).build(),
ChooserAction.Builder(
Icon.createWithResource(this, R.drawable.baseline_folder_copy_24),
"Add to folder",
PendingIntent.getBroadcast(
this,
1,
Intent(Intent.ACTION_VIEW),
PendingIntent.FLAG_IMMUTABLE or PendingIntent.FLAG_CANCEL_CURRENT
)
).build()
)
shareIntent.putExtra(Intent.EXTRA_CHOOSER_CUSTOM_ACTIONS, customActions)
startActivity(shareIntent)
With this in place, we’re now able to display custom actions within our share sheet, providing direct access to common actions for our application.
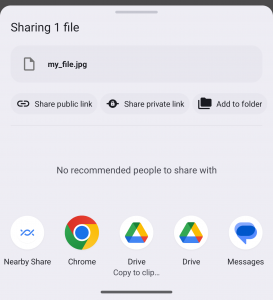
If you’re already supporting Android 14 in your app, these custom actions will be available for you to implement to provide users with a more streamlined sharing experience. I’m looking forward to seeing more apps adopting these actions in the apps that I use, please do share if you’re exploring these for implementation!