As of Compose Foundation 1.7.0-alpha07, the ClickableText composable has been marked as deprecated, with plans for it to be removed come 1.8.0. ClickableText is often used to handle URLs within text – while the composable handles the click event, we are still required to manually provide styling attributes via annotated strings.
To replace ClickableText, we instead need to utilise the LinkAnnotation class inside of the buildAnnotatedString function, allowing us to add the styling and functionality of links to text content – this functionality will also handle URL click events for us. In this post, let’s take a quick look at how we can migrate.
For current clickable text implementations, we’re going to see something like this in our applications:
ClickableText(
text = AnnotatedString("Open Link"),
onClick = { offset ->
// handle click
}
)
When migrating to the use of buildAnnotatedString, we will see our implementation transform to something like the following:
Text(buildAnnotatedString {
append("View my ")
withLink(LinkAnnotation.Url(url = "https://joebirch.co")) {
append("website")
}
})
Here we can see several steps:
- We use the buildAnnotatedString function to create a builder for our string
- We use append to add some initial text that is not to behave as a link
- We use the withLink function to push a LinkAnnotation onto our string, using the provided URL
- Within the body of the withLink function, we use append to push the string content that should be displayed for our link
It’s likely we’ve already used the buildAnnotatedString at some point in our projects already – its the addition of the withLink and LinkAnnotation here that power this with link functionality.
By default, our link will be displayed in our text as an underlined component:
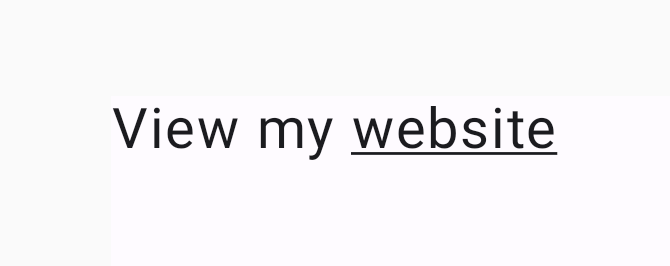
If we wish to change the styling of our linked text, we can pass a SpanStyle reference alongside the url. This allows us to control properties such as the color that is to be used for link.
Text(buildAnnotatedString {
append("View my ")
withLink(
LinkAnnotation.Url(
url = "https://joebirch.co",
style = SpanStyle(color = MaterialTheme.colorScheme.primary)
)
) {
append("website")
}
})
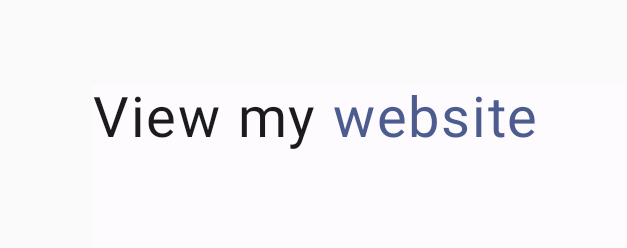
As we can see, migrating from ClickableText to LinkAnnotation is very little effort, allowing us to adopt a simpler and familiar API for highlighting links within our text. It’s advisable that you begin migrating once 1.7.0 hits stable, that way you are not blocked by any removals when it comes to future compose versions.